Python Built In Functions Cheat Sheet
This document is a quick cheat sheet showing how the PEP 484 typeannotation notation represents various common types in Python 3.
- From itertools import count, repeat, cycle, chain, islice. = count (start=0, step=1) # Returns updated value endlessly. = repeat ( , times) # Returns element endlessly or 'times' times. = cycle # Repeats the.
- Python functions are able to return multiple values using one return statement. All values that should be returned are listed after the return keyword and are separated by commas. In the example, the function squarepoint returns xsquared, ysquared, and zsquared. Def squarepoint (x, y, z): xsquared = x. x ysquared = y. y zsquared.
Python Built In Functions Cheat Sheet
Note
Python has a set of built-in functions. Abs Returns the absolute value of a number. All Returns True if all items in an iterable object are true. Any Returns True if any item in an iterable object is true. Cheatsheet for all. Built-In Functions. In Python, we have a function that calculate the product built-in to python. In Python, the definition of a function is so versatile that we can use many features such as decorator, annotation, docstrings, default arguments and so on to define a function. In this cheat sheet, it collects many ways to define a function and demystifies some enigmatic syntax in functions.
Technically many of the type annotations shown below are redundant,because mypy can derive them from the type of the expression. Somany of the examples have a dual purpose: show how to write theannotation, and show the inferred types.
Variables¶
Python 3.6 introduced a syntax for annotating variables in PEP 526and we use it in most examples.
Built-in types¶
Functions¶
Python 3 supports an annotation syntax for function declarations.
When you’re puzzled or when things are complicated¶
Standard “duck types”¶
In typical Python code, many functions that can take a list or a dictas an argument only need their argument to be somehow “list-like” or“dict-like”. A specific meaning of “list-like” or “dict-like” (orsomething-else-like) is called a “duck type”, and several duck typesthat are common in idiomatic Python are standardized.
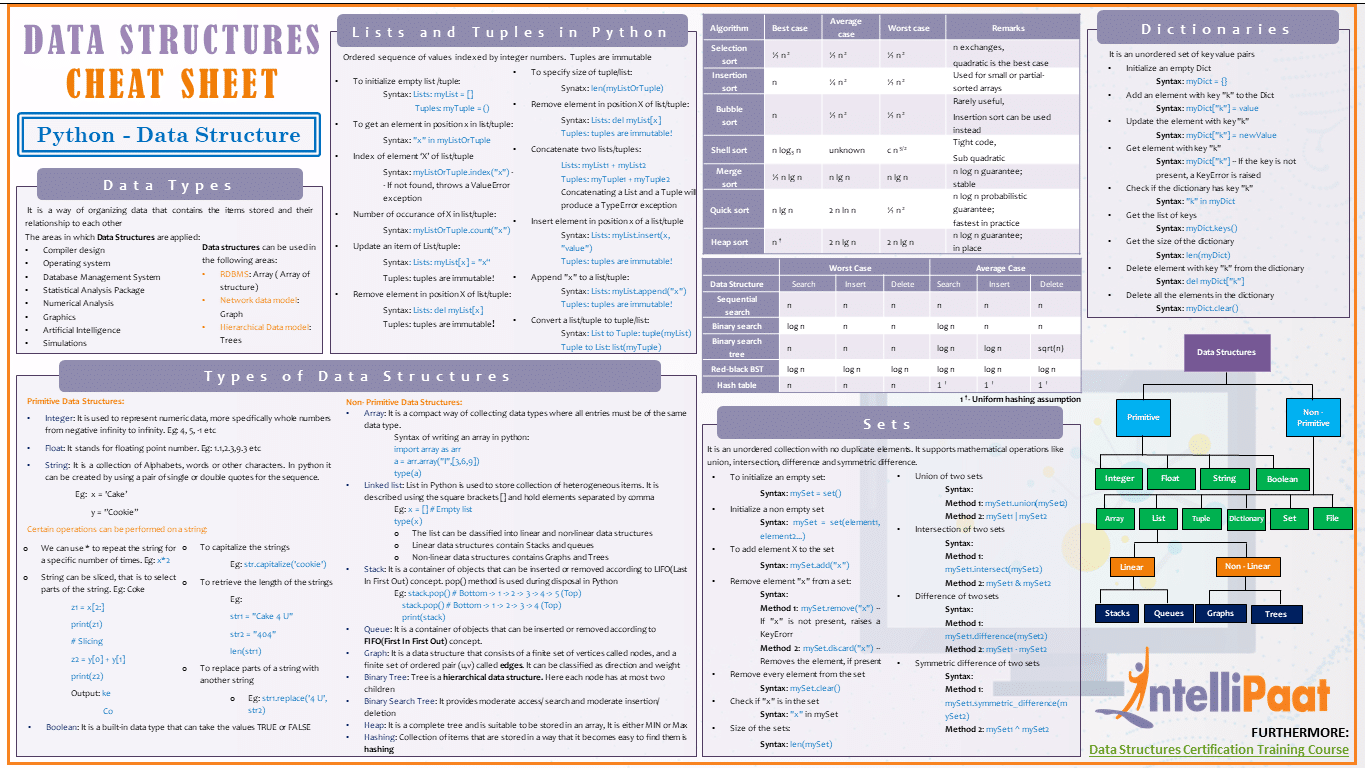
You can even make your own duck types using Protocols and structural subtyping.
Classes¶
Coroutines and asyncio¶
See Typing async/await for the full detail on typing coroutines and asynchronous code.


Miscellaneous¶
Decorators¶
Decorator functions can be expressed via generics. SeeDeclaring decorators for more details.
In Python, we have a function that calculate the product built-in to python.
See it all here : https://docs.python.org/3/library/functions.html
การที่จะตั้งชื่อฟังก์ชั่นนั้นไม่ได้ตั้งชื่ออะไรก็ได้นะครับ โดยชื่อฟังก์ชั่นที่ Python จองไว้ไม่ให้ตั้งชื่อเหมือน จะมีดังนี้ครับ
และเช่นเดียวกัน เราก็สามารถใช้ฟังก์ชั่นที่ Python ทำไว้แล้วได้เช่นเดียวกัน โดยการเรียกเหมือนกับฟังก์ชั่นธรรมดาเลย
abs() | dict() | help() | min() | setattr() |
all() | dir() | hex() | next() | slice() |
any() | divmod() | id() | object() | sorted() |
ascii() | enumerate() | input() | oct() | staticmethod() |
bin() | eval() | int() | open() | str() |
bool() | exec() | isinstance() | ord() | sum() |
bytearray() | filter() | issubclass() | pow() | super() |
bytes() | float() | iter() | print() | tuple() |
callable() | format() | len() | property() | type() |
chr() | frozenset() | list() | range() | vars() |
classmethod() | getattr() | locals() | repr() | zip() |
compile() | globals() | map() | reversed() | _import_() |
complex() | hasattr() | max() | round() | |
delattr() | hash() | memoryview() | set() |
# Using abs()
abs() is absolute in math language. Absoulte will returns a positive number from the value
# Using chr()
chr() converts number (integer only) to ASCII character.
# Using ord()
ord() converts single ASCII character to number (integer).
Python 3 Cheat Sheet
# Using len()
Mostly used with for loops. len is equal to length of something.
# Using max()
Function will return the largest number from 2 arguments
# Using min()
Function will return the smallest number from 2 arguments
# Using pow()
Caculates a exponential values by using functions. This is absolutely optional way to calculate exponent
# Using round()
Returns a number that will be rounded up (if >= .5) or round down (if < .5)
If you want to forced round up or round down, You can use math library to help
# Using sorted()
Returns a arrays of values that have been sorted, pending on their value types
Parameter reverse is when you want to sort in descending order
Parameter key is when you want to sort dictionary using only some data to determine
# Using chr() float() int()
Python Code Cheat Sheet Pdf
chr() convert value to string type (if possible)
float() convert value to float type (if possible)
int() convert value to integer type (if possible)
